1.BeanNameUrlHandlerMapping
The BeanNameUrlHandlerMapping
class maps URL requests to beans names. It is the default handler mapping class, so it is the one created by the DispatcherServlet
when Spring cannot find any handler mapping class declared. An example of using the BeanNameUrlHandlerMapping
class is shown below. There are two beans declared, the first one’s name is helloWorld.htm
and its class is the HelloWorldController
. So the BeanNameUrlHandlerMapping
will map any helloWorld
URL request to this Controller. The second bean’s name is the hello*.htm
and its class is also the HelloWorldController
. So, in this case, the BeanNameUrlHandlerMapping
will map any URL request that starts with hello
(such as helloWorld
, helloAll
) to the HelloWorldController
.
mvc-dispatcher-servlet.xml
09 | class = "org.springframework.web.servlet.view.InternalResourceViewResolver" > |
10 | < property name = "prefix" > |
11 | < value >/WEB-INF/</ value > |
13 | < property name = "suffix" > |
19 | class = "org.springframework.web.servlet.handler.BeanNameUrlHandlerMapping" /> |
21 | < bean name = "/helloWorld.htm" |
22 | class = "com.javacodegeeks.snippets.enterprise.HelloWorldController" /> |
24 | < bean name = "/hello*.htm" |
25 | class = "com.javacodegeeks.snippets.enterprise.HelloWorldController" /> |
So, check what happens when the calling the URL helloWorld.htm
:
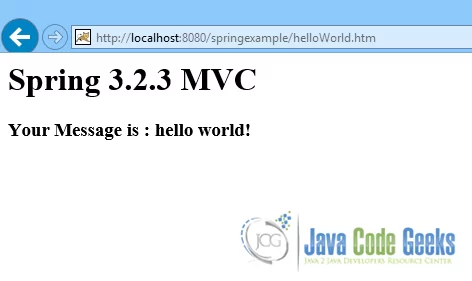
And here is the case of helloGeeks.htm
:
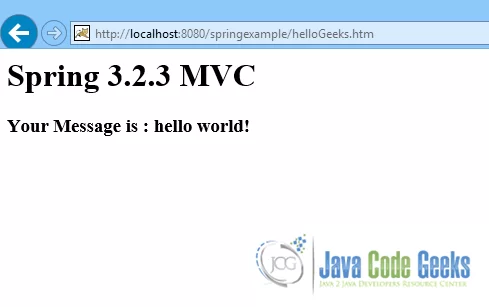
5. ControllerClassNameHandlerMapping
The ControllerClassNameHandlerMapping
class uses a convention to determine the mapping between request URLs and the Controller instances that are to handle those requests. In this case, there is no need to declare a bean name for the Controller. In the example below, the ControllerClassNameHandlerMapping
will map to the HelloWorldController
all URL requests that start with helloWorld
, or helloWorld*
. In the ControllerClassNameHandlerMapping
bean declaration there are two properties to configure, the caseSensitive
, which is set to true
, and the pathPrefix
, which is set to /javacodegeeks/
. These properties allow ControllerClassNameHandlerMapping
to also map to the HelloWorldController
all URL requests with uppercase characters, like helloWorldJavaCodeGeeks
, as also URL requests with path prefix like /javacodegeeks/helloWorld
.
mvc-dispatcher-servlet.xml
03 | class = "org.springframework.web.servlet.mvc.support.ControllerClassNameHandlerMapping" > |
04 | < property name = "caseSensitive" value = "true" /> |
05 | < property name = "pathPrefix" value = "/javacodegeeks" /> |
09 | < bean class = "com.javacodegeeks.snippets.enterprise.HelloWorldController" /> |
The cases described above are shown in the screenshots below.
Here is a case of uppercase characters:
And here is a case with a pathPrefix:
6. SimpleUrlHandlerMapping
The SimpleUrlHandlerMapping
provides a property called mappings
so as to be configured. This property is set in the bean declaration and consists of key value mapping pairs. It can be set in two ways, as shown below:
mvc-dispatcher-servlet.xml
02 | < bean class = "org.springframework.web.servlet.handler.SimpleUrlHandlerMapping" > |
03 | < property name = "mappings" > |
05 | < prop key = "/helloWorld.htm" >helloWorldController</ prop > |
06 | < prop key = "/*/hello.htm" >helloWorldController</ prop > |
07 | < prop key = "/hello*.htm" >helloWorldController</ prop > |
12 | < bean id = "helloWorldController" class = "com.javacodegeeks.snippets.enterprise.HelloWorldController" /> |
mvc-dispatcher-servlet.xml
02 | < bean class = "org.springframework.web.servlet.handler.SimpleUrlHandlerMapping" > |
03 | < property name = "mappings" > |
05 | /helloWorld.htm=helloWorldController |
06 | /*/hello.htm=helloWorldController |
07 | /hello*.htm=helloWorldController |
12 | < bean id = "helloWorldController" class = "com.javacodegeeks.snippets.enterprise.HelloWorldController" /> |
Note that the Controller
bean declaration uses an id
property, which is used in the SimpleUrlHandlerMapping
bean declaration for the mapping. Each one of the cases configured above, are shown in the screenshots below:
7. Handler mapping priorities
The handler mapping implementations described can be mixed and used together. The only thing that needs to be configured is the priority of each mapping class, so that Spring MVC DispatcherServlet
will know which handler mapping implementation to use with what priority. The priority can be set as a property in every mapping bean declaration, as shown below:
mvc-dispatcher-servlet.xml
02 | < bean class = "org.springframework.web.servlet.handler.SimpleUrlHandlerMapping" > |
03 | < property name = "mappings" > |
05 | /helloWorld.htm=helloWorldController |
06 | /*/hello.htm=helloWorldController |
07 | /hello*.htm=helloWorldController |
10 | < property name = "order" value = "0" /> |
13 | < bean id = "helloWorldController" class = "com.javacodegeeks.snippets.enterprise.HelloWorldController" /> |
17 | class = "org.springframework.web.servlet.mvc.support.ControllerClassNameHandlerMapping" > |
18 | < property name = "caseSensitive" value = "true" /> |
19 | < property name = "pathPrefix" value = "/javacodegeeks" /> |
20 | < property name = "order" value = "1" /> |
24 | < bean class = "com.javacodegeeks.snippets.enterprise.HelloWorldController" /> |
In this case, both ControllerClassNameHandlerMapping
and SimpleUrlHandlerMapping
are used, but the first one to handle a URL request will be the SimpleUrlHandlerMapping
.
No comments:
Post a Comment